Infinite Craft: Hacking on an infinite generative AI game
Follow @debarghya_dasInfinite Craft is yet another extremely addictive game made my one of my favorite creators on the internet, Neal Agarwal who publishes on his website, neal.fun. The game went instantly viral — Neal boasts a mind-boggling 1000 crafts / second and even 2 weeks after launch, he had 30,000 users in half an hour. The game creatively uses generative AI and large language models to allow players to craft an infinite set of things while starting with just 4 elements.
In this blog, I talk about how I wrote code to creatively explore the space of elements eventually synthesizing over 10,000 elements and discovering 1,000 for the first time!
Gameplay
Here’s how the gameplay works:
- You start with four elements — Water💧 , Fire🔥, Wind🌬️ and Earth🌍.
- You can combine two elements at a time which may or may not form a new element, based on a large language model. In this case, that is Facebook’s Llama 2 powered by Together.ai
- Elements combine in a fixed way for all players in the game. For example, Wind🌬️ + Fire🔥 = Smoke💨 for everyone. LLMs typically are not deterministic, so this is a design choice of the game. — When you synthesize a new element that no one in the world has seen before, it tells you it’s a First Discovery!
There’s really no point of the game other than to be amused at how things synthesize (Welfare + Homeless = Democrat), synthesize many and new elements, and get a kick out of discovering something for the first time. The space of elements to discover is near infinite. On the day of release, Neal posted that 200,000 elements had been crafted but not “Shake Shack”.
Programming
The first order of business for me was, of course, to figure out how to do this programmatically. Fortunately, the request was simple to reverse-engineer and the server always responded when the Referer
was set correctly. Here’s an example request:
curl -X GET "https://neal.fun/api/infinite-craft/pair?first=<word1>&second=<word2>" \
-H "Referer: https://neal.fun/infinite-craft/"
And the response looked something like this:
{
"result": "Wall Street",
"emoji": "🏦",
"isNew": false
}
You can put any two strings into the API and it takes about half a second to respond. Occasionally, the result
will be “Nothing” and isNew
denotes that you discovered something new that no one else has ever seen before.
I typically would not get rate-limited at around 2-4 QPS, but above that Cloudflare would block my IP for several hours.
Searching Possibilities
Breadth First Search: Building the base
The first thing I wanted to do was to try all possible combinations of 2 elements and progressively expand to see how far we can go. In Computer Science terms, this is called breadth first search. With this approach, we can exhaustively get up to 4 levels deep:
- Level 0: 4 total elements
- Level 1: 10 total elements, 6 possible combinations, 6 created, 100% fusion rate
- Level 2: 30 total elements, 45 possible combinations, 20 created, 44.4% fusion rate
- Level 3: 137 total elements, 435 possible combinations, 107 created, 24.6% fusion rate
- Level 4: 1020 total elements, 9316 combinations, 883 created, 9.5% fusion rate
The next level expansion would be long and cumbersome, because (1020 choose 2) in combinatorics leaves 519,690 possibilities! At this point, we decide to try something different. Here’s a graphical visualization of the first 2 levels:

Beam Search: Finding new elements
After I explored up to level 4, I still hadn’t discovered new elements. I figured I’d try a different strategy where I’d pick a sample of words, iterate through a bunch of random combinations and then use the new created element set to keep creating more elements. Sometimes, I’d inject specific starter words to try to find specific concepts.
Eventually, with this approach, I quickly started discovering new elements. The fusion rate converged at 10-15% and about 10% of those created “New” concepts. Although most of the new concepts were frankenstein words like Ghost Rattlesnake + Steamlantis = Steam Rattlesnake and Fart + Stingart = Stingfart, I was proud of being the first to discover Chana Masala.
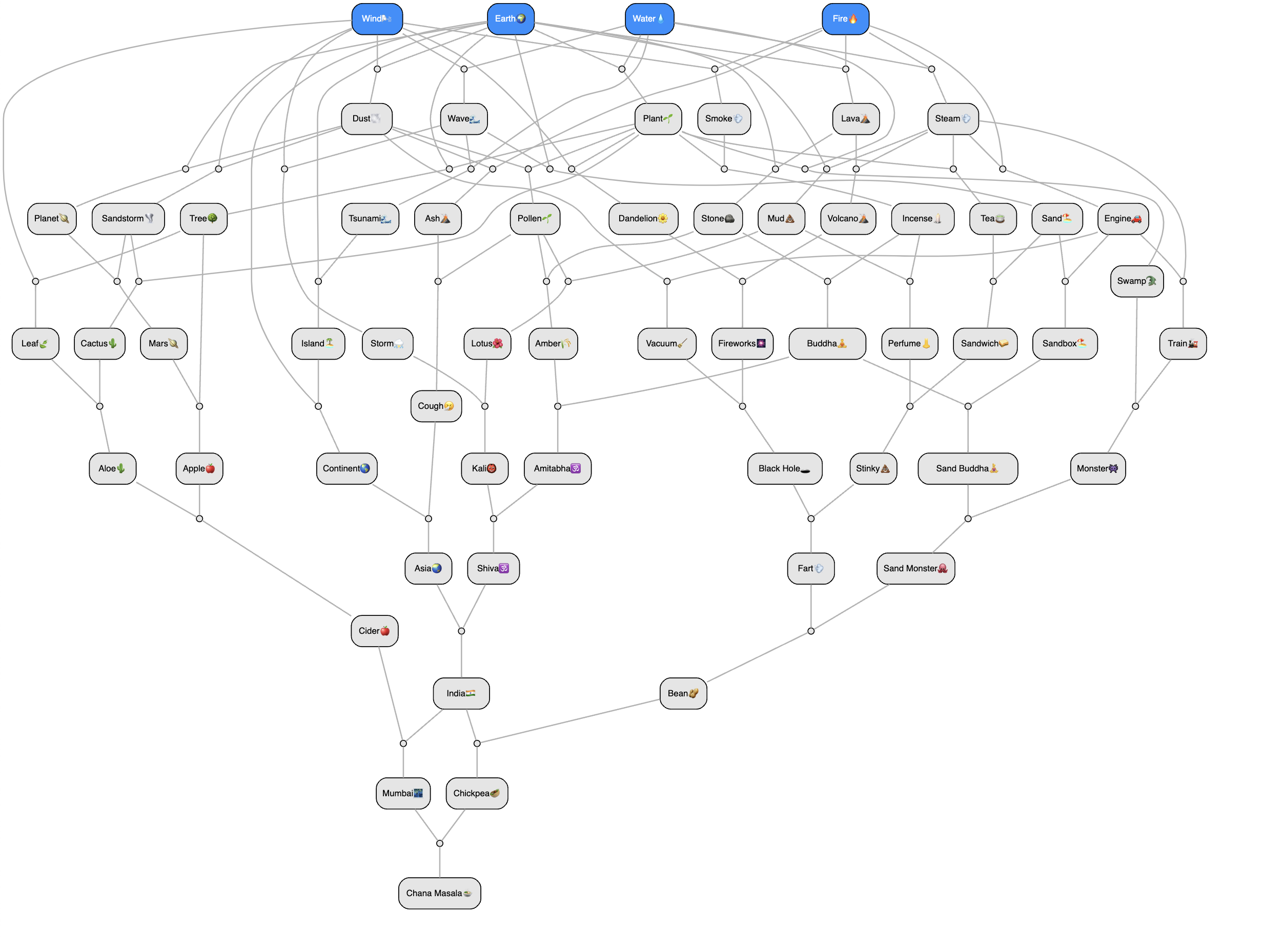
While this one is quite tractable visually, there are some discoveries that are far more complex. Of course, this may not be the quickest path to generating that element.
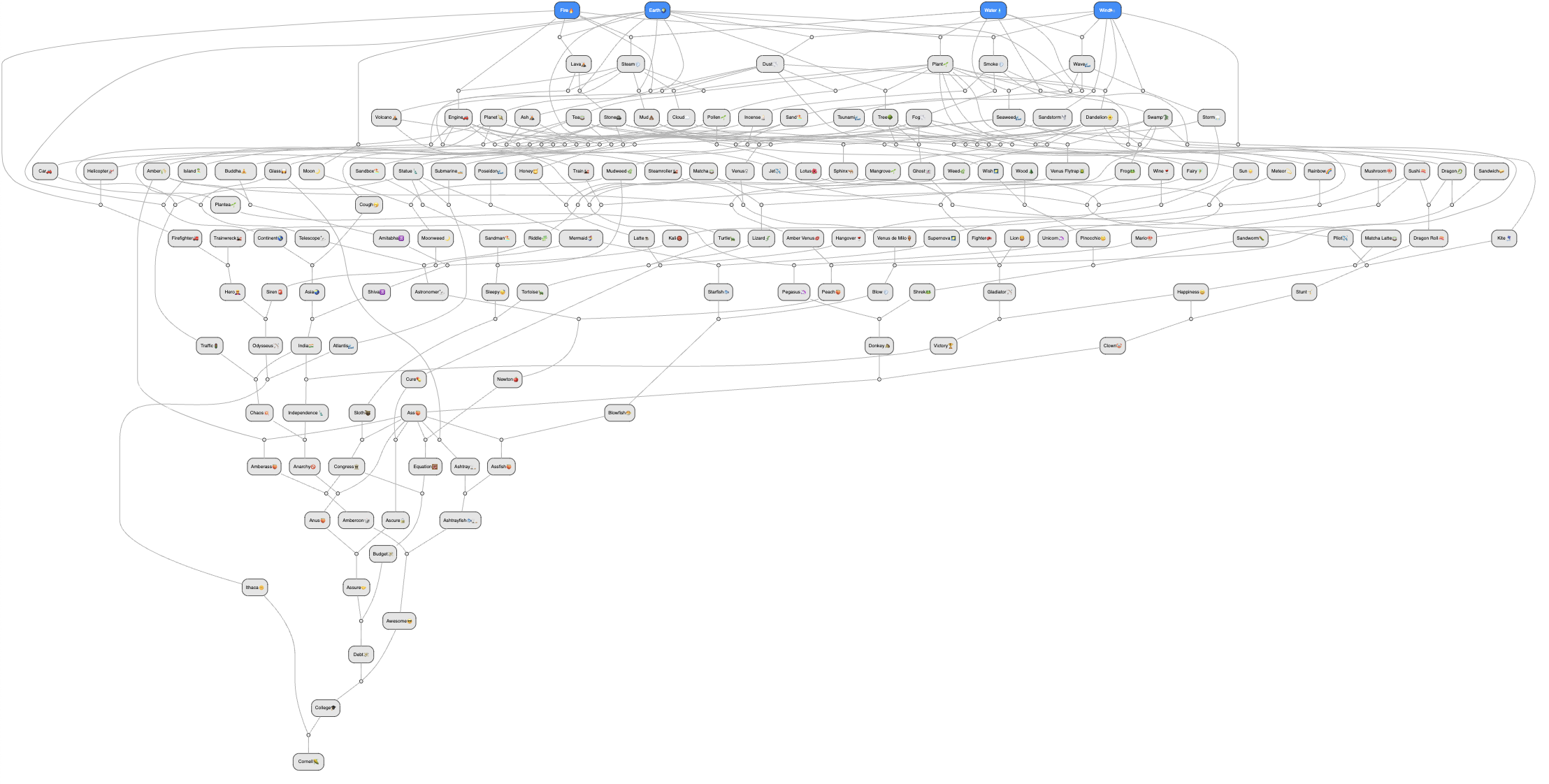
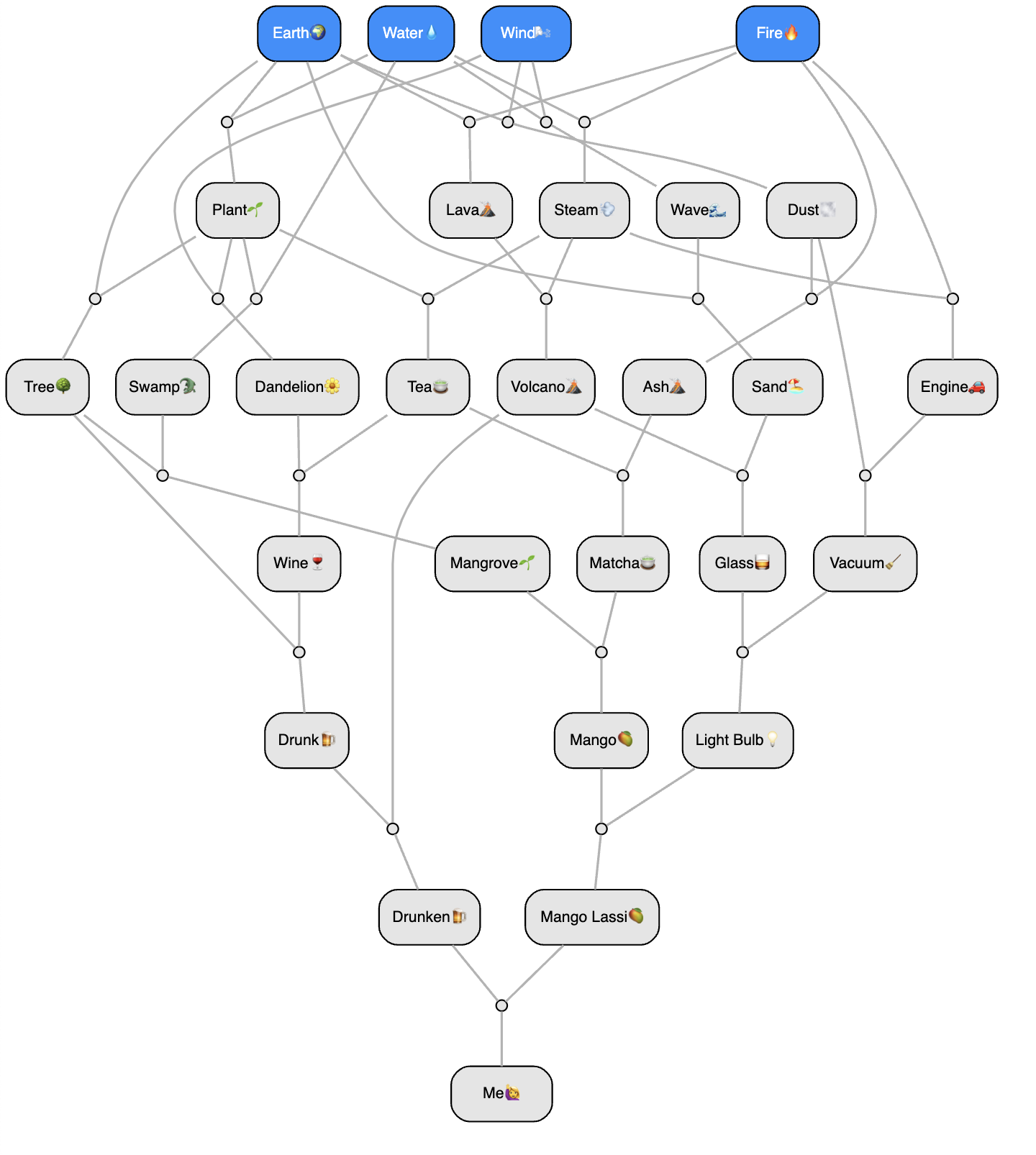
In the end, I discovered over 10,000 elements and 1,000 elements for the first time! Here are some of the funnier combinations:
- Yoyo + Asia = Yoyoyoyoyoyoyoyoyoyoyoyoyoyoyoyoyoyoyo
- Sugar + Ghee = Halwa
- DNA + Artichoke = Artificial Intelligence
- Funny + Wall = Facebook
- Mirth + Anus = Hemorrhoid
- Latin + Corruption = Catholic
- Friend + Shame = Friendzone
- Hacking + Homework = Cheating
- Rubber + Conspiracy = Condom
- Hacktivism + Suicide = Aaron Swartz
- Computer + Speech = Siri
- Harvard + Math = MIT
- Ithaca + College = Cornell
- Hacktivist + Google = Googleplex
- Drunken Submarine + Banana = Banana Boat
- Mango Lassi + Drunken = Me
And here are a couple of notable first-time discoveries:
- Gnocchi + Contraception = No Gnocchi
- Cricket + Bengali = Ganguly
- Colorado + Urinal = Colo-RAD-o
- Racist + Ayurveda = Ayuracist
- Epidemic + Tandoor = Naandemic
- Delhi + Jobs = Deloitte
- Soup And Surf And Turf + Lord of the Rings = Lord Of The Rings, Soup And Surf And Turf
- Chutney + Harry Potter = Chutney Potter
- Road Rage + Chitty Chitty Bang Bang = Chitty Chitty Bang Bang Bang Bang Bang Bang Bang
- Edible + Paint = Food Coloring
- Mumbai + Chickpea = Chana Masala
Why?
Exploratory global exploration games like this are some of my favorite kind of programming challenges because you get to use all kinds of unconventional techniques to get better. It reminded me of some similar projects / puzzles I did in the past:
- Divergent Association Task: Trying to guess 10 words that are the most different from each other to maximize the score.
- How close can you get to the hash? I don’t remember the details, but in college there was a global challenge to find inputs that would SHA256 hash to as close to the target values as possible. Because you’d register via email addresses, colleges could team up against each other and its a compute race to see who can get the closest hash.
- Wordle Solver
- 2048
Huge shout out to Neal for making such a fun, creative game.
I love hearing feedback! If you don't like something, let me know in the comments and feel free to reach out to me. If you did, you can share it with your followers in one click or follow me on Twitter!